Introducing Hibernate
Hibernate is a Java framework that simplifies the development of Java application to interact with the database. It is an open source, lightweight, ORM (Object Relational Mapping) tool. Hibernate implements the specifications of JPA (Java Persistence API) for data persistence.
Steps for using Hibernate Framework
1:Create a Basic Database(in Derby)
2:Create new project using hibernate framework
3:Create pojo class
4:Create .hbm.xml file
5:Edit .hbm.xml file
6:Make a form and a corresponding servelt
7:Check working of code
Create a Basic Database(in Derby)
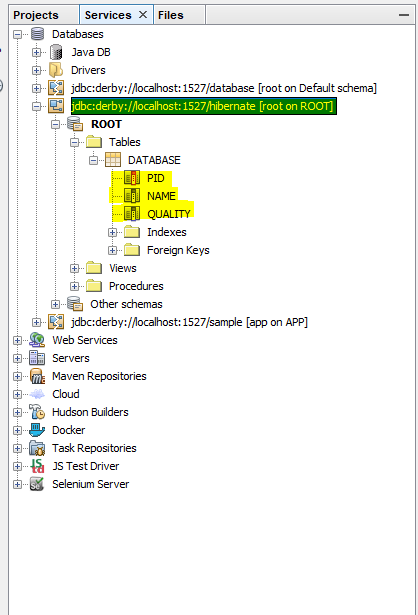
1:Go to service and Right click on Database and create a new database . 2:Right click and then execute command . 3:There create table with attributes you want.
Create a new project with hibernate framework
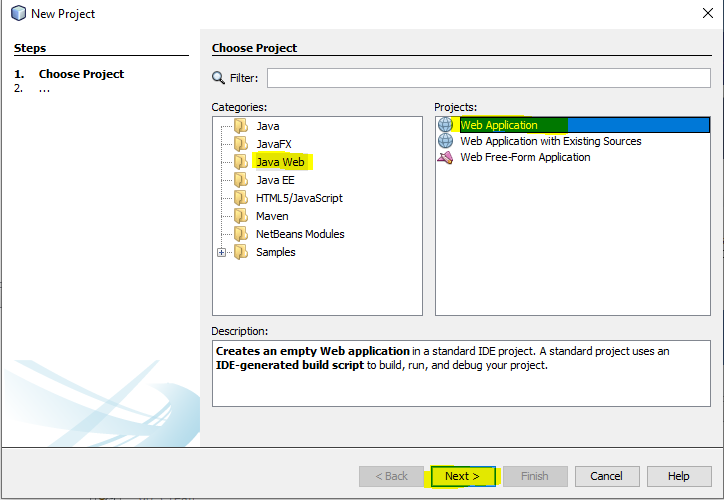
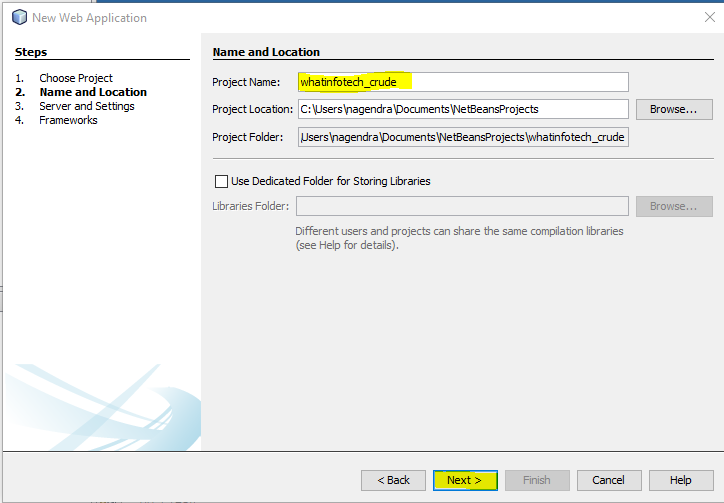
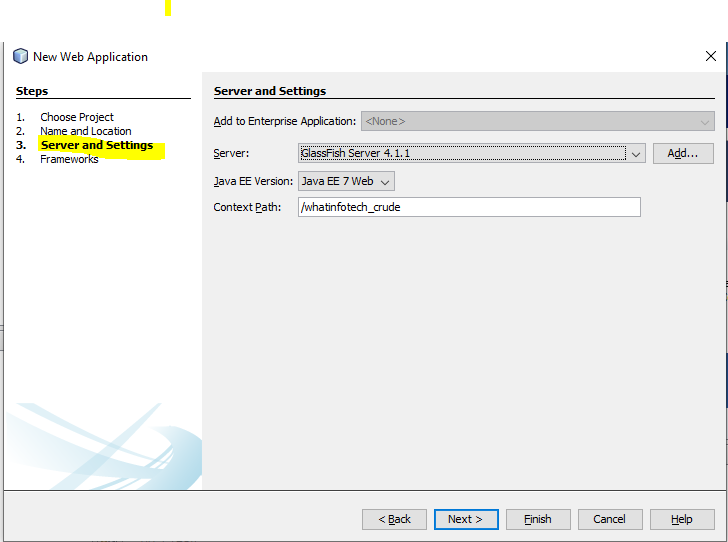
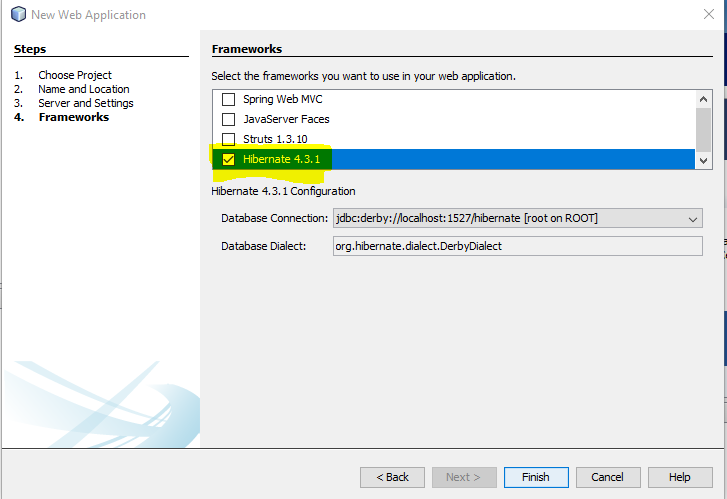
For this Step we need to first make a Database and connect to it.
For Connecting go to Services -> url of Database -> Right click -> Connect
No need to create hibernate.cfg.xml because netBeans does it for us.


hibernate.cfg.xml
<hibernate-configuration>
<session-factory>
<property name="hibernate.dialect">org.hibernate.dialect.DerbyDialect</property>
<property name="hibernate.connection.driver_class">org.apache.derby.jdbc.ClientDriver</property>
<property name="hibernate.connection.url">jdbc:derby://localhost:1527/hibernate</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">root</property>
<mapping resource="hibernate.hbm.xml"/>
</session-factory>
</hibernate-configuration>
Creating a Pojo class
Pojo class in a java class which contains the setter and getter methods for the attributes which are in table.

First right click on Project and create a new java file.
Then Define the variables same as the attribute in the table.
Then Right click and Click insert code -> getter() and setter()

Then the pojo class will be ready.
Database.pojo /** * @author nagendra */ public class database { int pid,quality; String name; public int getPid() { return pid; } public void setPid(int pid) { this.pid = pid; } public int getQuality() { return quality; } public void setQuality(int quality) { this.quality = quality; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
Creating a .hbm.xml file
It is a mappping file.
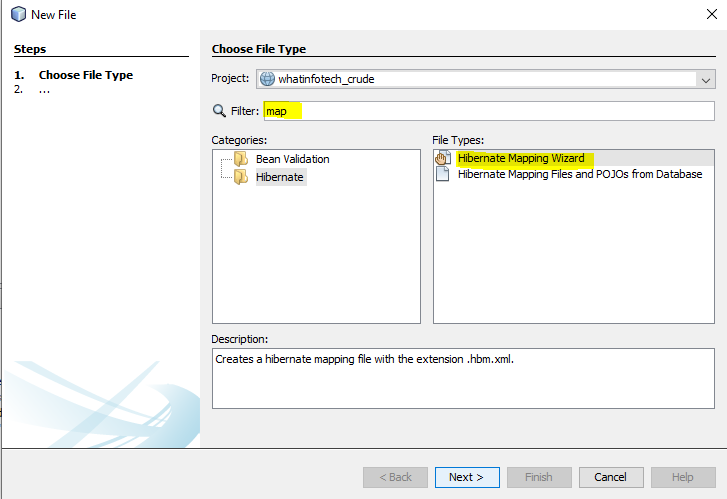
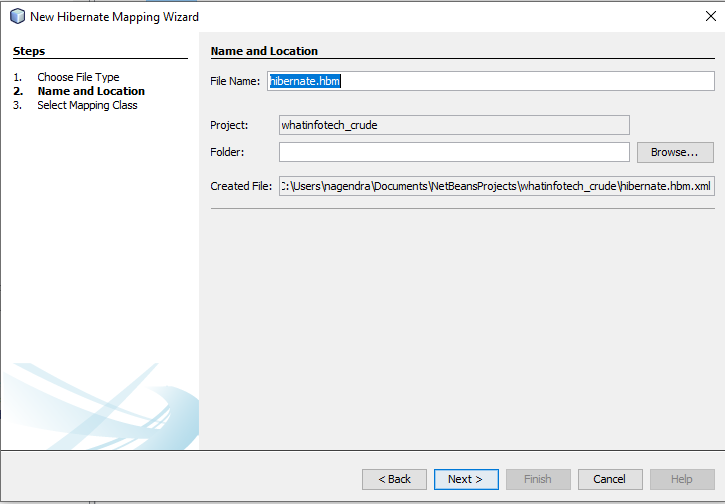
Editing .hbm.xml file
After creating the mapping .hbm.xml file now we need to edit it .

Remove the closing tags from class attribute and map each of the attribute of table and the pojo class variables.
NOTE:Try to give same names so that the mapping becomes easy.
<hibernate-mapping>
<class name="database" table="DATABASE" >
<id name = "pid" column = "pid"></id>
<property name = "name" column = "name" />
<property name = "quality" column = "quality" />
</class>
</hibernate-mapping>
Create a basic form in HTML and Corresponding Servelet
index.html
<!DOCTYPE html>
<!--
To change this license header, choose License Headers in Project Properties.
To change this template file, choose Tools | Templates
and open the template in the editor.
-->
<html>
<head>
<title>Crud application</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<div>
<form action="action">
PID :<input type="text" name="pid" value="" />
NAME:<input type="text" name="name" value="" />
QUALITY:<input type="text" name="quality" value="" />
<input type="submit" value="submit" />
</form>
</div>
</body>
</html>
action.java
import java.io.IOException;
import java.io.PrintWriter;
import static java.lang.System.out;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
/**
*
* @author nagendra
*/
public class action extends HttpServlet {
@Override
public void doGet(HttpServletRequest rq, HttpServletResponse rs){
try{
rs.setContentType("text/html");
int pid =Integer.parseInt(rq.getParameter("pid")); //getting parameters from front end
int quality=Integer.parseInt(rq.getParameter("quality"));
String name=rq.getParameter("name");
PrintWriter out =rs.getWriter();
database w = new database(); //creating pojo class object
w.setPid(pid); //calling setter method to insert data
w.setName(name);
w.setQuality(quality);
try{
Configuration c;
c = new Configuration().configure("hibernate.cfg.xml"); //creating configuration object
SessionFactory s1= c.buildSessionFactory();
Session s = s1.openSession();
s.save(w); //saving the data
s.beginTransaction().commit(); //committing the changes
out.println("Success");
}
catch(HibernateException ee){
out.println(ee.getMessage()+"Inside the Configuration try catch");
}
}
catch(IOException | NumberFormatException | HibernateException e) {
out.println(e.getMessage());
}
}
}
Working of code

Checking the database
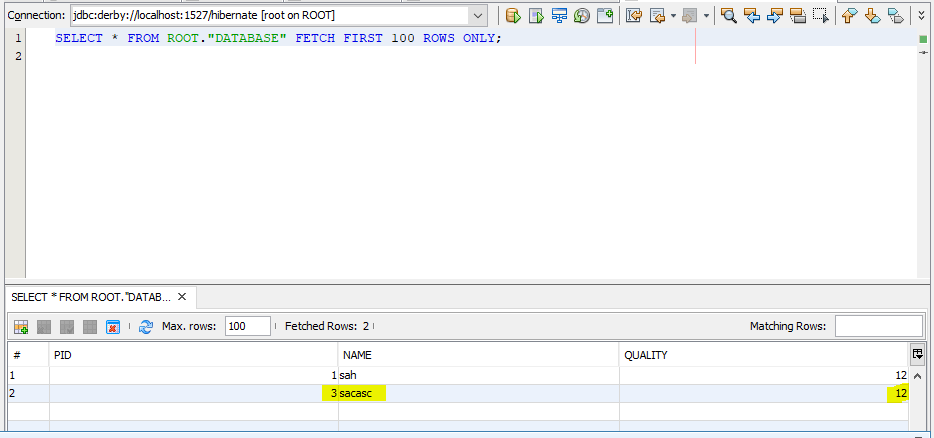
The Database is updated so it means that connectivity is Done properly.
THE CORRECT FILE STRUCTURE IS
